MGTK
Themable GUI framework.
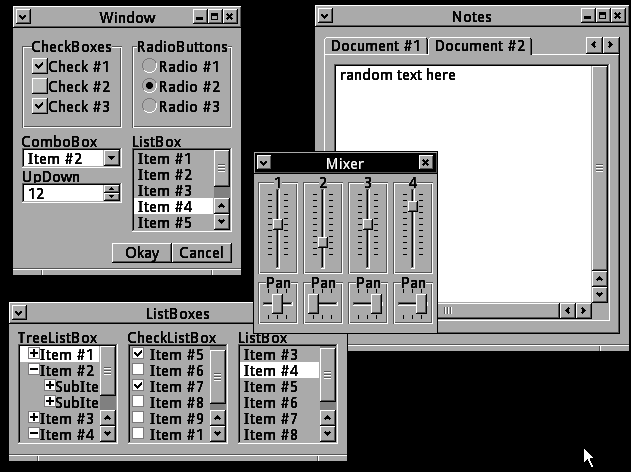
I’ve always enjoyed a lot those old DOS games that implemented windowing UI elements. I’ve spent countless hours playing SimCity, Transport Tycoon, Theme Park and the like as a young kid. When I started making games I ended up gravitating towards creating similar games.
I’ve played a bunch with Allegro’s Atari ST’s-inspired windowing system, back when I was making games in C. When I started using c# at work and migrated to XNA/MonoGame for my hobby gamedev projects I didn’t find any tools or libraries that satisfied my UI needs at the time.
I was using WinForms a lot at work projects, so I ended up building MGTK inspired by that structure and philosophy.
public class frmMixer : Form
{
public frmMixer(WindowManager windowmanager)
: base(windowmanager)
{
WindowManager = windowmanager;
int grpWidth = 40;
for (int i = 0; i < 4; i++)
{
GroupBox grpChannel = new GroupBox(this)
{
X = i * grpWidth + (i + 1) * 5, Y = 0,
Width = grpWidth, Height = 100,
Text = (i + 1).ToString()
};
TrackBar tckVolume = new TrackBar(this)
{
Parent = grpChannel,
X = 10, Y = 15, Width = 20, Height = 80,
TickStyle = TickStyle.Both,
Orientation = TrackBarOrientation.Vertical,
BackColor = new Color(170, 170, 170)
};
grpChannel.Controls.Add(tckVolume);
Controls.Add(grpChannel);
GroupBox grpPan = new GroupBox(this)
{
X = i * grpWidth + (i + 1) * 5, Y = 100,
Width = grpWidth, Height = 50,
Text = "Pan"
};
TrackBar tckPan = new TrackBar(this)
{
Parent = grpPan,
X = 5, Y = 15, Width = grpWidth - 10, Height = 30,
TickStyle = TickStyle.Both,
Orientation = TrackBarOrientation.Horizontal,
BackColor = new Color(170, 170, 170),
Maximum = 2
};
grpPan.Controls.Add(tckPan);
Controls.Add(grpPan);
}
this.MaximizeWindowButtonVisible = this.MinimizeWindowButtonVisible = false;
}
}
Code to create the Mixer form pictured above